Deploying RAGs in Production: A Comprehensive Guide to Best Practices
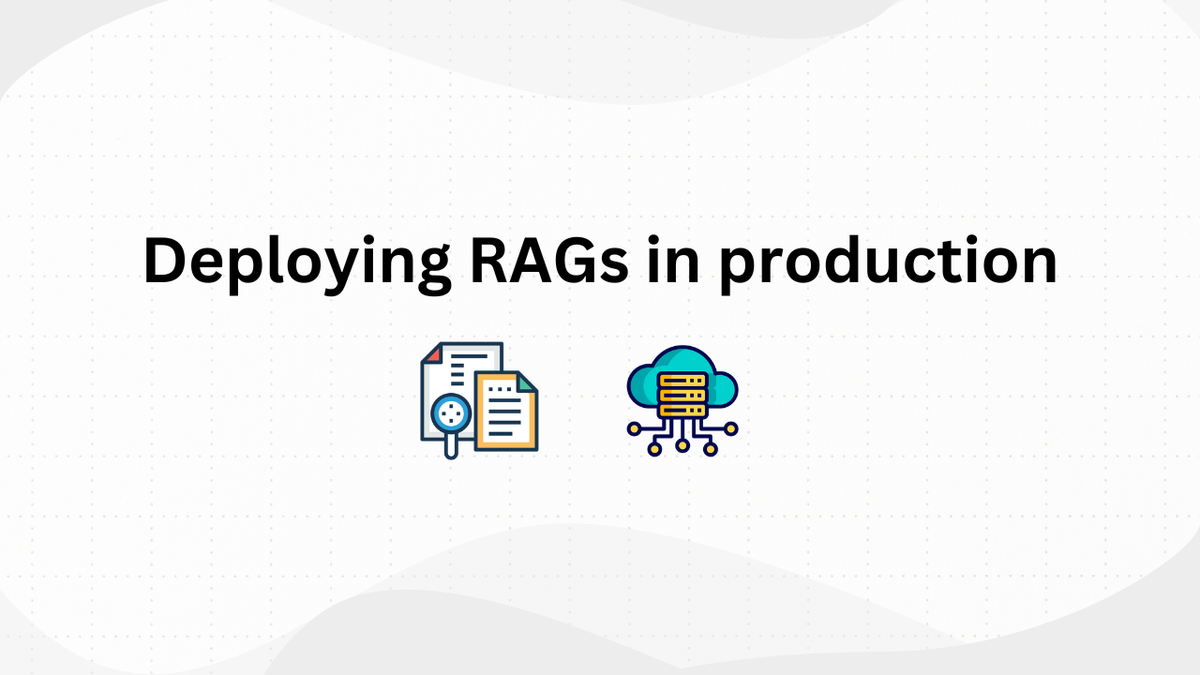
Retrieval Augmented Generation (RAG) systems are transforming the AI landscape by blending the accuracy of retrieval methods with the creative power of Large Language Models (LLMs). This combination ensures that AI responses are both precise and contextually relevant by grounding them in factual data. This guide provides actionable insights for AI development teams, helping you build robust, scalable, and effective RAG systems.
By the end of this guide, you will learn how to:
- Optimize data ingestion and preprocessing
- Implement effective retrieval strategies
- Overcome common challenges in RAG deployment
- Deploy and monitor RAG systems effectively
Core Components of a RAG System
A modern Retrieval-Augmented Generation (RAG) system consists of three main pipelines:
- Indexing Pipeline: Ingests and preprocesses data, creates vector embeddings, and stores them in a fast vector database for easy retrieval.
- Retrieval Pipeline: Fetches relevant information from the indexed knowledge base using combined retrieval strategies and re-ranking techniques.
- Generation Pipeline: Combines retrieved data with user queries to produce clear, accurate, and contextually appropriate responses.
Understanding these components is essential for building an effective RAG system. Each pipeline plays a key role in ensuring the system delivers accurate and relevant answers.
Best Practices for RAG Deployment
Deploying a RAG system successfully requires careful attention to various aspects, including data management, embedding strategies, retrieval optimization, generation processes, and deployment practices. Below are the best practices to follow:
1. Data Management and Preprocessing
Accuracy in RAG deployment starts with clean, structured, and context-rich data. Establish strong preprocessing workflows to ensure high data quality and relevance.
- Clean Data Pipeline: Correct errors, annotate metadata, and remove duplicate content. Use domain-specific filters to enhance the data's semantic richness. Implement a Data Quality Funnel Model to cleanse and structure data systematically, ensuring only high-quality, relevant information is used. This reduces noise and inconsistencies, boosting AI performance.
- Hierarchy Maintenance: Keep the structural hierarchy of documents (like titles and sections) to improve chunking accuracy and context coherence. During preprocessing, use the prefixing technique by adding descriptive text segments at the beginning of each data chunk. This enhances both retrieval and generation quality, especially for large documents.
- Advanced Chunking Strategies: Use rolling windows and hierarchical boundaries for smooth content segmentation. During preprocessing, apply element-based chunking to divide documents by structural elements (e.g., headings, paragraphs). This ensures that chunks contain only relevant data, improving retrieval and generation by large language models.
Code Example: Advanced Document Parsing with Element-Based Chunking
from langchain_community.document_loaders import PyPDFLoader
from langchain_text_splitters import RecursiveCharacterTextSplitter
def parse_and_enrich(file_path, chunk_size=500, chunk_overlap=50):
loader = PyPDFLoader(file_path)
documents = loader.load()
text_splitter = RecursiveCharacterTextSplitter(
chunk_size=chunk_size,
chunk_overlap=chunk_overlap,
separators=["\n\n", "\n", " ", ""]
)
chunks = text_splitter.split_documents(documents)
return chunks
2. Embedding and Vector Management
Embeddings are crucial for any RAG system, turning text into vector representations that capture semantic meaning.
- Domain-Specific Fine-Tuning: Customize embedding models like
SentenceTransformers
to improve accuracy in specific domains. Recent advancements show that making embedding models aware of the retrieval context can enhance performance. Contextual document embeddings adjust vector representations based on the retrieval context, leading to more accurate results. For example, the Tabular Embedding Model (TEM) fine-tunes embeddings for tabular data in RAG applications, improving performance in specialized areas. - Scalable Vector Databases: Use advanced vector databases such as Pinecone or Weaviate to enable fast, high-precision operations across large datasets. Vector databases store embeddings and support similarity searches, allowing quick retrieval of related information. They are essential for managing and querying vectorized data in RAG systems.
- Metadata Integration: Enhance embeddings with metadata for advanced filtering and better contextual understanding during retrieval. Adding metadata like document type, author, or publication date can improve the precision of retrieved information, especially in complex RAG applications.
Code Example: Domain-Specific Embedding Pipeline
from sentence_transformers import SentenceTransformer
import pinecone
class EmbeddingPipeline:
def __init__(self, model_name, pinecone_api_key, environment, index_name):
self.model = SentenceTransformer(model_name)
pinecone.init(api_key=pinecone_api_key, environment=environment)
self.index = pinecone.Index(index_name)
def generate_embeddings(self, texts):
embeddings = self.model.encode(texts, convert_to_tensor=True)
return embeddings
def upsert_embeddings(self, ids, embeddings):
vectors = list(zip(ids, embeddings))
self.index.upsert(vectors=vectors)
3. Retrieval Optimization
Effective retrieval ensures that the most relevant information is sent to the generation pipeline, directly enhancing system performance.
- Hybrid Retrieval Models: Combine lexical (e.g., BM25) and vector-based search strategies to improve recall and precision. For example, Elasticsearch can be configured to use hybrid models by mixing text relevance scoring with dense embeddings from pre-trained models. Weaviate offers built-in hybrid search capabilities, allowing fine-tuning parameters to balance semantic and lexical weights. These configurations optimize retrieval precision for complex queries.
- Re-Ranking Techniques: Use advanced cross-encoders, such as BERT-based models, to re-rank retrieved results more accurately by scoring their semantic alignment with the query. This not only refines the top results but also ensures contextual relevance by considering query-specific details. Models like ColBERT or Sentence-BERT can significantly enhance retrieval precision in complex queries, as shown in datasets like MS MARCO and TREC Deep Learning benchmarks.
- Dynamic Query Reformulation: Improve queries by rephrasing or breaking them into simpler sub-queries for better recall and precision. For instance, sub-query decomposition splits a complex query into multiple simpler ones, each targeting a specific aspect of the user's intent. Tools like Elasticsearch support query boosting and fine-grained filtering, while libraries like Transformers can generate paraphrased queries dynamically to increase retrieval diversity and precision.
Code Example: Hybrid Retrieval Pipeline
import weaviate
class HybridRetriever:
def __init__(self, client_url):
self.client = weaviate.Client(client_url)
def hybrid_search(self, query, top_k=10):
results = self.client.query.get("Document", ["content", "metadata"]) \
.with_hybrid(query=query, alpha=0.5) \
.with_limit(top_k) \
.do()
return results
4. Generation Pipeline
The generation stage is where RAG systems turn retrieved information into useful insights.
- Grounded Prompts: Use advanced prompt engineering to keep model outputs tied to the provided context. Techniques like context tagging and retrieval-grounded completion ensure that generation tasks are linked to retrieved data, reducing hallucinations. Align tokens with contextually relevant embeddings for higher factual accuracy.
- Output Compression and Prioritization: Use ranking algorithms like Reciprocal Rank Fusion (RRF) or contextual scoring to evaluate and prioritize retrieved chunks by relevance. Techniques such as late interaction models (e.g., ColBERT) refine chunk prioritization by leveraging query-document interactions for better contextual alignment. Additionally, dimensionality reduction methods (e.g., PCA or UMAP) can compress input vectors while retaining their semantic meaning, improving computational efficiency and response quality.
- Few-Shot Learning: Improve response accuracy using example-driven prompts with domain-specific scenarios. Techniques like in-context learning allow for adaptable query resolution, while tools like LangChain and OpenAI's APIs support structured prompt examples, ensuring consistency across specialized queries.
5. Deployment and Monitoring
A production-ready RAG system must be resilient, scalable, and adaptable to changing data landscapes. Effective deployment and continuous monitoring are key to maintaining system performance and reliability.
Kubernetes Orchestration
Kubernetes is essential for deploying scalable and fault-tolerant RAG systems. It helps manage resources efficiently, scale your system as needed, and ensure high availability.
Key Steps for Kubernetes Deployment:
- Define Deployments and Services: Use YAML files to define your RAG system’s deployments and expose them as services.
- Implement Auto-Scaling: Set up Horizontal Pod Autoscalers (HPA) to adjust the number of pods automatically based on the system load.
- Monitor Health: Use readiness and liveness probes to monitor pod health and restart unhealthy pods automatically.
- Use Secrets and ConfigMaps: Secure sensitive information like API keys with Kubernetes Secrets and manage non-sensitive configurations with ConfigMaps.
Monitoring with LangSmith
LangSmith is a comprehensive developer platform that simplifies every step of the application lifecycle, from logging to observability. It integrates easily with RAG systems, providing robust monitoring with minimal setup.
Key Features of LangSmith:
- Cross-Provider Logging: Supports multiple LLM providers (OpenAI, Azure, Anthropic, etc.).
- Customizable Metadata: Enables detailed tracking through fields like run_name, project_name, session_id, and tags.
- Advanced Observability: Offers a centralized view of performance metrics such as response times, error rates, and system usage.
LangSmith offers a user-friendly interface for tracking and analyzing RAG system performance, making monitoring straightforward and effective.
Monitoring with Athina
Athina is an advanced monitoring platform designed specifically for LLM-powered applications. It provides real-time monitoring, detailed analytics, and easy evaluations, making it ideal for enhancing RAG system performance and reliability.
Key Features of Athina for RAG Monitoring:
- Cross-Provider Logging: Supports multiple LLM providers (OpenAI, Azure, Anthropic, etc.).
- Metadata Segmentation: Tracks extensive metadata fields such as prompt_slug, customer_id, and expected_response for detailed insights.
- Context Logging: Logs retrieved-context for RAG applications, helping track the quality of retrieval and generation.
- Plug-and-Play Integration: Easy integration using callbacks, reducing setup time.
Athina provides a comprehensive monitoring solution, offering detailed insights into your RAG system's performance and making troubleshooting efficient.
Ensuring Scalability and Reliability
To maintain scalability and reliability, Kubernetes orchestration should be combined with strong monitoring practices. Key considerations include:
- Auto-Scaling: Continuously assess workloads and use Kubernetes HPA to manage traffic spikes effectively.
- Health Checks: Define liveness and readiness probes to ensure quick recovery and system availability.
- Resource Allocation: Optimize resource usage with Kubernetes’ resource limits and requests to avoid contention.
- Data Freshness: Automate updates to your knowledge base to keep your RAG system delivering current and relevant results.
By integrating these practices, you ensure that your RAG system is not only robust but also adaptable to changing workloads and evolving data needs.
Case Studies of Successful RAG Deployments
Examining real-world examples of successful RAG deployments provides valuable insights and inspiration, demonstrating how organizations have effectively leveraged RAG systems to enhance their operations.
1. PepsiCo
Overview:
PepsiCo integrated RAG-enabled LLMs to optimize supply chain management and enhance market analysis capabilities.
Key Focus Areas:
- Security and Efficiency: Leveraged robust data governance and access control mechanisms to ensure data security and system efficiency.
- Scalability: Implemented scalable infrastructure to handle large volumes of data and user queries, ensuring consistent performance.
Outcomes:
- Enhanced Decision-Making: Improved accuracy and relevance of market insights, enabling more informed decision-making processes.
- Operational Efficiency: Streamlined supply chain operations through accurate and timely data retrieval, reducing operational costs and improving efficiency.
2. JetBlue
Overview:
JetBlue deployed RAG-enabled LLMs publicly, focusing on robust security measures to protect user data and prevent misuse.
Key Focus Areas:
- Infrastructure Enhancement: Utilized Databricks on Azure to enhance the management and deployment of their RAG LLMs, providing the necessary infrastructure for large-scale data processing and AI model integration.
- User Data Protection: Implemented stringent security protocols to safeguard user data, ensuring compliance with data protection regulations.
Outcomes:
- Improved Customer Support: Enhanced the accuracy and relevance of customer support responses, improving overall customer satisfaction.
- Scalable Deployment: Achieved a scalable deployment model capable of handling high traffic volumes without compromising performance.
3. Korea Press Foundation
Overview:
In collaboration with Upstage, the Korea Press Foundation developed a news-specialized generative AI solution using RAG. This system conducts real-time news-based searches and responses without hallucination concerns, leveraging a vast dataset of news articles and advanced search and query engines.
Key Focus Areas:
- Real-Time Data Handling: Enabled real-time access to the latest news information, ensuring that responses are current and relevant.
- Advanced Search Engines: Implemented sophisticated search and query mechanisms to enhance the accuracy and relevance of retrieved information.
Outcomes:
- Reliable Information Retrieval: Achieved high levels of accuracy in news information retrieval, minimizing the risk of hallucinations and ensuring factual correctness.
- Enhanced User Engagement: Improved user engagement through timely and relevant news-based responses, fostering greater trust and reliance on the system.
Conclusion
Deploying Retrieval-Augmented Generation (RAG) systems in production involves careful planning and execution across several components, including data management, embedding strategies, retrieval optimization, generation processes, and deployment practices. By following the best practices outlined in this guide, you can build a robust, scalable, and efficient RAG system capable of delivering accurate and contextually relevant AI responses.
Additionally, using advanced monitoring tools like LangSmith and Athina ensures that your RAG system remains performant and reliable as it scales and adapts to changing data landscapes. Embrace these practices to fully leverage the potential of RAG systems and drive impactful AI-driven solutions in your organization.